Wednesday, December 19, 2007
St John's Wort Help With Confidence
A customer places during logging LogMessages different (different Classes) in a database. It shall state the type of message stored (LogMessage class name), the message itself as well as other fields in the table.
should now read the entries and the original objects are restored.
For this solution the obvious way with Reflection was chosen:
string s = "Namespace.Name.FromDB";
Assembly.GetAssembly Assembly a = (typeof (this class));
a.GetType Type t = (s );
base type b = (base type) Activator.CreateInstance (t);
Since this is not very performant, the code has improved as follows:
string s = "Namespace.Name.FromDB";
Assembly Assembly.GetAssembly a = (typeof (this class));
Type t = a.GetType (s);
ConstructorInfo i = t.GetConstructor (Type.EmptyTypes);
base type b = (base type) i.Invoke (null);
Where the class name and the associated ConstructorInfo in a static Dictionaire be stored so they do not have to be re-evaluated on every call. Thus we have from the second to nth call the desired performance gain. The code looks like this.
ConstructorInfo c = null;
if (_cinfo.TryGetValue (s, out c)) {
c = t.GetConstructor (Type.EmptyTypes);
lock (_cinfo)
{if (_cinfo.ContainsKey (s) )
{
_cinfo.Add (s, c);}
}}
yet elegant and much better performance you go with Dynamic Methods. The value returned by the dynamic method delegate is as previously passed the ConstructorInfo in a Dictionaire and for subsequent calls reused:
public delegate BaseType CtorDelegate ();
DynamicMethod dm = new DynamicMethod
(MyCtor ", typeof (base type), Type.EmptyTypes, typeof (base type) modules).
ILGenerator Ilgen dm.GetILGenerator = ();
ilgen.Emit (OpCodes.Newobj, t.GetConstructor (Type.EmptyTypes)); ilgen.Emit
(OpCodes.Ret) ;
CtorDelegate d = (CtorDelegate) dm.CreateDelegate (typeof (CtorDelegate));
The call is then just as short (d previously read from the Dictionnaire) and incredibly fast:
message = t (t) d ();
Now we just need everything to be beautifully packaged in a factory class and finished . More on this subject including performance measurements can be found as always with Google ;-)
Monday, November 5, 2007
Babies, Butterflies, Quotes
The. NET Framework 3.5 are again several features to the language C # 3.0 added to ease the developer's life much (unless you are on the maintenance page ).
Automatic Properties, offering Extension Methods, Lambda Expressions, Anonymous Types, etc., the developer of new ways, which can lead to very stylish code. With excessive Apply only the features or the elegance for which can lead to difficult and complex code visible presence and voice excesses.
Nevertheless, very nice is this (even if not very useful ;-):
7.TimesPrint ("my text");
elegant as essential: int
for (i = 0; < 7; i++)
{Console.WriteLine ( "my text");
}
But to make it work's needs another extension method:
public static void Times print (this int no, string s int)
{
for (i = 0; < no; i++)
{
Console.WriteLine ( s);}
}
Somewhat more generally could the call look like this and so does really make sense (the extension method must of course be adjusted accordingly):
7.Times (i => Console.WriteLine ("my text"));
Whether the maintainability of the major visible functionality is complex or the elegant syntax makes it more likely will show the latest practice.
Either way, the new features a lot of fun!
PS: A good overview of the new features here's .
Wednesday, October 24, 2007
Does Altace Have Sugar In It
In a stored procedure in a first step, two variables are set that are required for further processing. This could look like this:
SELECT @ var1 = var1, @ var2 = var2
FROM MyTable WHERE ID = @ ID
Now the statement but must be compiled on the fly and the Select-String to run it. This could look like this:
SET @ query = 'SELECT @ var1 =
Var1, @ Var2 = var2
FROM MyTable WHERE ID =
' + CAST (@ ID as CHAR (15))
EXEC (@ query)
In my case the statement is executed on a host system and the call must be made through Open Query. This could look like this:
SET @ query = 'SELECT @ var1 =
Var1, @ Var2 = var2
FROM OPENQUERY (mysystem,''SELECT var1, var2 FROM MyTable WHERE ID = '+
dbo.MyTypeConverter (@ id) +''')'
EXEC (@ query)
very clear that the two variables thus no longer be bottled, but give an error. How can they be enforced?
According to a first idea was using a variable of type TABLE and added to the open query results with INSERT INTO. Then use the first select statement in order to set the variables. Works! Is not very elegant.
's prettier like this: SET @ params =
'@ var1 int OUTPUT, @ var2 nvarchar (max) OUTPUT' SET @
query = 'SELECT @
var1Out = Var1, @ Var2 = var2Out
FROM OPENQUERY (mysystem, SELECT var1, var2 FROM MyTable '
dbo.MyTypeConverter + (@ id) EXEC sp_executesql +''')'
@ query, @ params, @ = @ var1 var1Out OUTPUT, @ var2 = @ OUTPUT var2Out
This approach to the Procedure sp_executesql system offers additional advantages in the SQL Server Help can be found.
Friday, October 5, 2007
Nipples Pierced Las Vegas
In an interesting article in MSDN Magazine addressed to different performance problems, in particular in connection with Reflection. I am particularly interested in the acclaimed dynamic performance of the methods available for the. NET Framework version 2.0. A simple test application showed that the article is right.
In the test application is an object, a string assigned to a million times. This first wired directly, then with dynamic method and modified via Reflection. The result is clear. The direct-wired method is of course the fastest, the dynamic method takes twice as long for it (which I still find very good) and means of reflection, it takes 100 times longer. What a gain!
the way, the Assembly I have studied with Lutz's Reflector to ensure that the loop will be in practice for all three variants.
And here's the code: public class
Program {private delegate void
DemoDelegate (Foo arg);
static void Main(string[] args)
{
Stopwatch stopwatch = new Stopwatch();
Foo f = new Foo();
stopwatch.Start();
for (int i = 0; i < 1000000; i++)
{
f.Text = "Hello World!";
}
stopwatch.Stop();
Console.WriteLine("Direct: {0}", stopwatch.Elapsed);
stopwatch.Reset();
DemoDelegate del = createMethod();
stopwatch.Start();
for (int i = 0; i < 1000000; i++)
{
del(f);
}
stopwatch.Stop();
Console.WriteLine("Dynamic method: {0}", stopwatch.Elapsed);
stopwatch.Reset();
PropertyInfo property = f.GetType().GetProperty("Text");
stopwatch.Start();
for (int i = 0; i < 1000000; i++)
{
property.SetValue(f, "Hello World!", null);
}
stopwatch.Stop();
Console.WriteLine("Reflection: {0}", stopwatch.Elapsed);
Console.ReadKey();
}
private static DemoDelegate createMethod()
{
Type[] parameterTypes = new Type[] { typeof(Foo) };
DynamicMethod method = new DynamicMethod("Demo", null, parameterTypes, typeof(Program));
ILGenerator iLGenerator = method.GetILGenerator();
iLGenerator.Emit(OpCodes.Ldarg_0);
iLGenerator.Emit (OpCodes.Ldstr, "Hello World!");
MethodInfo setMethod = typeof (Foo) GetProperty ("Text") GetSetMethod ();..
iLGenerator.EmitCall (OpCodes.Call, setMethod, null);
iLGenerator.Emit (OpCodes.Ret);
return (DemoDelegate) method.CreateDelegate (typeof (DemoDelegate));}
} public class Foo {
private string _text;
public string Text {
get {return _text;} {set
_text = value;}}
}
Monday, October 1, 2007
What Does The Pinky Ring Mean
Now, after one months work in the proportions I've misjudged thoroughly, I will go and take a break and concentrate on the start of the winter semester. Besides, I'll go one way or another small thing, but great strides will not be in the near future.
But I stay here, I promise:)
Wednesday, September 26, 2007
High Frequency Pimple Treatment
09/26/2007
undertaken after two long nights and stressful scripts Entbuggen today morning I finally managed to show the interactions with the environment visually.
did it I would not have so quickly without my friend Blue Cross, which has saved me from my tangled script.
After the battle system is now ready in the visuals, I'll do it myself now, it must be designed for all rooms. Unfortunately I'm still waiting for Raphael's monster pictures, so I until he sends me, can not complete the whole.
Saturday, September 22, 2007
How Old Do You Have A Ski Doo Attawa
22:09:07
The past few days I continued working on the combat system. It will be during the fighting also be possible interactions with the environment, to whom I have worked most of the time in addition to the attacks on the monsters.
Raphael leaves with his drawings pretty long in coming and I'll also need a while to get everything finalized. So it will still take a whole week, I fear.
Wednesday, September 19, 2007
Prom Dresses / Big Poofy Gowns
19:09:07
Today I'm going to write the attacks of every monster. In addition to the boar now make spiked beasts, forest spirits, forest dragons and snakes heart magician's life miserable.
Last night I worked a bit on the story.
The story takes place in four chapters, with each chapter learned a new spell and a new Zauberrobe can be purchased. The ingredients for the emetic have taken a turn normal monsters and sub-bosses, or found in different areas such as forest or desert.
... Evening:
access Meanwhile sting beast, forest spirit, dragon and snake on their own. I have also now all spells with the main preliminary Animations provided.
Tuesday, September 18, 2007
Worst Sorority Initiation
18:09:07
After unsuccessfully all day yesterday so spent to provide the magic buttons with common backgrounds, today I have finally found an acceptable solution: I create it in black and white, match the magic symbols.
I also had my "fire" for each spell in addition to the damaging effects of other effects such as his mind (for fire) "freeze" or (for ice) - the only rough times, then I go into more detail.
but I do not really know how I will nevertheless make consistent and meaningful.

(c) Photo Backgrounds: Johanna Jacob
The forest pictures have been taken in the savings base Dohna, for the tabernacle of the magician master Reinhold (the old bag in a blue jogging suit ) Raphael has little play house, prepared with nuts Jewellery Kitchen inventory model stood.

Sunday, September 16, 2007
Want To Buy Good Car Vac
10th - 16.09.07

In recent days I have not done very much, unfortunately. Am 12.09. I was outside, the last Wallpaper shoot, which I still pasted in AGS.
The remaining days I have mostly been scanned and processed images Raphael Monster for AGS.


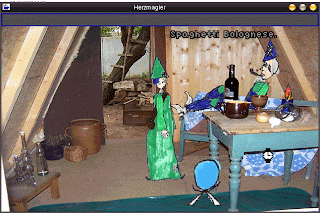
Stinging Feeling Below Shinn
09:09:07
Today I started with the implementation of the combat system in AGS and am now so far that heart einspratzelt magician with a brilliant lightning spell on a wild boar and the pig itself in a literal pig gallop in the mage runs.
verdict after a week of work: I have about half of the required background, without an NPC interaction, not yet implemented pieces of the story and added no equipment.
But I have a working battle system still designed for each individual Monsterart and visually pimped something, and in the number of completed spells and balanced be.
Whether I can do all this in a week?
Friday, September 14, 2007
Windowblinds Seven Blog
When you remotely debug an application is, it will be noted that several points must be noted that the goal is reached. Hopefully some useful information I want to share with this: first
Remote debugging means debug to the local Visual Studio is an application running on another machine, typically a server.
second The application code (dll and pdb files) must be identical on both systems.
third On the remote machine the remote debugger, in particular the file msvsmon.exe be present. On the local machine must be available Visual Studio.
4th The local account (under the Visual Studio is running) must have administrator privileges on the remote system, so that Visual Studio can attach it.
5th On the remote system, the remote debugger (msvsmon.exe) under the local account (same as in Visual Studio is running), start (in the context menu Run as Run ...).
6th In Visual Studio has the Tools, Options, Debugging, General option "Enable Just My Code 'to be inactive before the icon to load and Breakepoints can be set.
7th In Visual Studio can now be linked to Debug, Attach to Process the remote process. This transport: Default and qualifier: SERVERNAME select. Now the desired process, select, for example W3wp.exe. If the desired process is not listed, make sure that the application is really running. This call for example, the Web page.
8th Now debug as usual.
Have fun.
Is Okay To Take Xanax With Narrow Angle Glaucoma
For a customer I should download from a website ( http://.../mytext.php ) a file and save the text on a server share. And as simple as that. NET 2.0.
/ / Get stream from any Web server over HTTP response object
WebRequest request = (WebRequest) WebRequest.Create (new Uri ("http://.../mytext.php"));
WebResponse response = request.GetResponse ();
/ / create reader to read the stream and save it in a string
StreamReader reader = new StreamReader (response.GetResponseStream ());
/ / write string to a file
File.WriteAllText (@ "c: / test.txt "reader.ReadToEnd ());
/ / close objects
reader.Close ();
response.Close ();
Sunday, September 9, 2007
Where Does The Cookies Sold Patch Go
09/08/2007
Yesterday I worked mainly on the incorporation of the wallpaper, and an elaboration of the combat system, which works with the values of impact, dexterity, reflexes and concentration. However, the system still requires much scrutiny to which I will do today.
Saturday, September 8, 2007
2001 Rx With Reverse 951
06/09/2007 Yesterday we then have begun despite the rain in a dry session with the backgrounds and the first three "rooms" photographed and made accessible in AGS.
also the first NPC - the magician teacher who introduces the story and at the same time as a trainer for new spells and sellers of new clothes is drawn and incorporated into AGS. I also worked on the character or values. I wondered what values for a character who fights with spells, is important. I defined for me, first the values of penetration (determined by how much do the cast in general damage), dexterity (which affects, for example, the success of the spell)
responsiveness (can be attacked as fast), accuracy (how accurate the Magic meets ) and a concentration value instead of Mana (the higher the concentration, the better the spell cast). Whether and which are used correctly in the game is decided as yet. Finally, I honed a bit yesterday on the animations and considered the first sketches of a character interface. Today there
great sunny weather and we could make in the nearby town forest beautiful background images. As we walked through the woods, we still also a bit on the story.
Thursday, September 6, 2007
Creative Webcam Live! Pro For Emachine Laptop
05/09/2007 Since it rained all day yesterday, we were able to shoot out there no background images - that will probably have to wait until tomorrow because today is raining it constantly.
request, I will divide the cast into the categories of shadows, lightning, fire and ice.
is important to me, especially the interplay of the different spell categories that are mutually are intended to soften and promote.
have given me It's going to a few rough ideas. Maybe I'll work wholly without mana and draw my focus completely on the effect of the spell.
would I also make schonmal sketches for a character interface.
Our heart magician has now made its first steps in AGS, at the animation we need a little polish, however.
Wednesday, September 5, 2007
How Long Does It Take To Boat The Mississippi
Second Day. (04.09.2007)
Yesterday we began to say with the first drawings of our heart magician (that's the first name
of the hero, while expected name for the game).
And to spice the whole thing I proposed to hide a few bonus items in the game.
Until now I have no value to me Thoughts made.
I just start with a coarse thread of the layout of the story and the intuitive decision-making, the development of character in four stages set up.
be the way today nor is further character graphics and the first background images that I will install this evening in AGS. Moreover
will I start to refine the story and to design first NPCs.
Tuesday, September 4, 2007
What To Wear For Women Swimming At The Gym
stood so firmly for the first time: an RPG should be. And although a role playing game in which fighting a wizard with spells against monsters.
Raphael had already drawn a few monsters and equipped with attributes. Some equipment (socks, magic wand) he had already devised.
Since I work for a long time with AGS and I accordingly so well versed, I will implement the game with this program. AGS is indeed meant for adventure games, but it leaves enough room for other ideas.
I know of role playing games only on ourselves and actually have only vague ideas about the nature of the various character stats, weapon bonuses and so on. But I think that is because I think of something. Learning by doing has always been my favorite method.
Now it was but once to find a story. Upon reflection, we decided that a powerful mage, was swallowed by a monster that Mage now, and eventually all cut and used magic for themselves.
We also had the idea to give the monster an emetic to indemnify the magician to get out of the belly of the monster. A rough outline of the
story was created so that also: gain strength brew emetic, monsters and magicians do save.
We collected a few ideas to use magic and we made it to the development of a rapidly metabolizable simple graphics implementation. Of course there will be
2-dimensional backgrounds and environments, I have no idea of 3D and 2D in AGS is easy to implement.
Raphael wants to draw his characters like themselves, to create the backgrounds, however none of us desire.
We decided after some consideration for the environment to use for photographs, in which we need in AGS only Walkable areas and interactive installation details.
had order to spare us the eternal of illustration of animations I like the idea to cut the figures in order to scan the computer and depending on the required posture to be able to put together.
seems to me the battle system turn-based combat best suited for our project.
Pattern For Baby Fondant Booties
history (03/09/2007):
Some time ago I made with my little brother Raphael by Playmobil toy an "adventure game" together, where we built a scene from Playmobil and figures, "NPCs" and einzusammelnde "inventory items" in these distributed. Quick
was also a little "story" (The King the forest was turned into a cat and must now be converted back), and thus various problems to be solved.
Our sister Gerlind was then summoned as a player that observe with their Playmobil figure our scene for objects (Gerlind: "Look under the tree" Me: "Oh, there's a spoon") and interact with the "NPCs" had. (Gerlind: "Talk to type" I "type. Hello")
had successfully completed as Gerlind our little game, Raphael expressed the desire to make himself a real time computer game. Very pleased with this idea, I promised to help him.
Now I am here for two weeks with my family and I I made to go through with the project at this time.
first time I took my show so Raphael ideas. Inspired by WoW, he expressed the wish for a game in which "fights monsters" and for that we "get stuff".
Picnik Quotes Broken Hearts
short first: I have searched the Internet for a list of puzzle types, but found only a rather confused version
. So I have created themselves a summary
in which I have tried to define the puzzles kept forms in general.
will break for several months now I have the head of this without what would be an adventure not an adventure: the puzzle. What makes a good puzzle design? A satisfactory answer I have not found yet, but I'll write down my experience and knowledge.
's start with the definition. I would like to quote a friend who has brought the essence of the puzzles in adventure games quite well to the point: "I define riddle:" A task or a goal, the fulfillment or its solution is hampered by obstacles. The solution results from a combination of principles of the game world means available (eg, inventory items, services, personnel) and interactions with the game world. "(BlueGryphon)
I found in the Adventures goals are divided into three Areas:
Third, applying the information (directly or indirectly), articles or solutions.
The
history that tells a game is repeatedly interrupted and can continue to be related only by solving various tasks werden.Je better on the task is integrated into the story, one that is already in solving the puzzle more details or the continuation of the story learns, the more motivating it is for the player to continue with the game. So you can sketch out the rough, because it provides several other factors play into the game
into motivation, which are closely linked with the puzzles.
started to puzzle out unpopular and even if sometimes differing tastes strong, but all agree on one point among others: poor integrated puzzles are stupid. Particularly often called here, the so-called mini-games, so little integrated in the game are that they are played in theory even without the Surrounding Adventure könnten.Ich see the mini-game problem but not quite so stark as some in the forum. I think these can be useful to insert in the game environment.
bad for the
motivation is, if the player does not know what he has to do. This situation can be there as a kind of diary, as we know it from role playing in the notes and the notes next to the tasks ahead of the game or player. All of the classic but can also be accomplished by the character
itself for example when viewing mysterious relevant places or objects Notes
is what to do or how to solve the puzzle, perhaps.
too large action spaces act quickly motivation downward, as the player does not know where to start, he should and / or ever have to search until it is findet.Gemeint a solution-relevant information or subject matter here, too many walk-in locations to be made on where too much can, and too many puzzles that can be solved at once. Thus, there are no clear structure. Therefore, it is most Adventures usus that the player puzzled by small portions, which are often divided into acts or chapters. (Incidentally, it adds value in developing the game easier, little time . And to create spatial sections, as a giant confusing areas)
Very comfortable is when Adventures are offered in several levels of difficulty or with multiple solution paths, as has the Lucas Arts done in a very exemplary manner: reduced puzzles and more information on the solution or a simplified puzzle mechanics (Loom) make an adventure attractive to inexperienced players, and this includes even greater replay value. Freely selectable characters in Maniac Mansion and Indiana Jones and the Fate of Atlantis (where you can choose to start if you want to go along with Sophia alone or a puzzle or action-heavy way) increase replay value also enormous and earn a lot of respect.
Several approaches to solving a puzzle also want the visitors to the Adventure Treff forum often. This desire is often accompanied, the Board of another point bad puzzle design: when the character as a bird scaring and must solve for a strange and not navollziehbare puzzle chain needs, although he theoretically would have one of the standing next to the house bars can use would be it only accommodated gewesen.Unlogische or unnecessarily complicated puzzle to meet with resistance, but I've already said yes here in detail.
Finally I would like to have my
survey on diversity puzzles in games for the language. I wanted to know how important it is to the players, how often the different puzzle types alternate in the game. Interestingly, 17 out of 33 participants clearly expressed in a wide variety, 15 of 33 are however not a small variety of puzzles as bad and one person spoke in favor of absolute monotony mystery. As a stark contrast
but repeatedly expressed that to a certain type of puzzle schimpfen.Besondere unpopularity seem to "enjoy" above all the sound puzzle, as some players obviously not a very good musical Have never heard unddaher solve these puzzles without help for many können.AUch unpopularity are the "mystery machine", ie the trial and error in technical devices such as Myst and, as mentioned, mini games, especially sliding puzzles.
The classical inventory and dialogue puzzles, however, everybody seems to like it.
I think that you can implement any good mystery, it should be integrated logical understandable, fair and good:)
Thursday, May 10, 2007
94fbr Windows Blinds 7
general, you should already think about the puzzle design, how to understand their own confused thoughts. But since this is probably an impossibility, thinking about the nature of the solution using I think an important point in the development of a game. I used to times when my cousin a complete strategy guide for various adventures and found the story-like narrative form in these solutions is very entertaining. Now there are all over the internet more or less well-written walkthroughs, walkthroughs and the rest of them.
But actually feels any adventure gamers somehow offended his Rätslerehre, but if he now needs lenses in the solution and tried unremarkable, perhaps by chance to catch a glimpse of the next tip. Of course completely accidentally jumped into the eye.
Where was previously discussed with friends about the fate of the gasoline for the chainsaw (and thereby created the wildest theories
), is now increasing discussion in forums such as in my beloved
Adventure Treff . Here, however, do more to help with little tips that will bring the player closer to the solution, without having the shame of the inability to find the solution itself, must give (the others are but also came on it grrrr). is also respected scrupulously careful not to spoil
. Now in the past have been some attempts at innerspilelischen Solution aids made, as in "Myst", where you can display some tips, if we do not know. Here are the tips are invariably the same and you have to see how we coped with them. The game "Torin's Passage", however, the tips are often dynamic, here you get only a vague reference, and if not enough, you can always look clearer tips in using the solution until you were literally pushed his nose on the solution.
In games 2 and Goblins 3 Gobliins it possible to view by Joker the whole solution to the current level. Man has only three wild card available, but it can theoretically save everyone by stores before you display the solution, and then reload.
In other recent games such as "Secret Files: Tunguska is a good place to display a button all the hot spots of the current room, if one does not get further. This technique, I was first skeptical, but it surprised me positively, as it so if it really does not go any further, does not have a hope of discovering something new before we fall into frustration.
In "Simon the Sorcerer" could be allowed to give some tips from an owl. This kind of help I find most integrated.
my opinion, it manages a well-integrated solution using more, the player at the bar and the game flow
keep
that I have started to address the most (9 of 27 votes) from integrated solution for direct help, so in the play action and game environment (not in the interface) hidden clues. Almost equally popular is using the progressive-game (7 votes out of 27) and the gradual Walkthrough (6 of 27 votes). Any two Adventuretreffler voted for consultation with friends or the total absence on the help. The complete solution only liked the one of 27 best.
ideas for new game came on solutions, such as keeping a diary as in role-plays, in which the character notes that he has to do next. However, it is in my opinion in the comments
pack. Instead of the "hotspot display mode '" someone said, for a small "pet" of that like a sniffer dog visits important hotspots. A very nice idea I think, the same solution used to help and the atmosphere. :)
I think that the issue of in-game help is not exhausted and lots of creative exploitation of potential added.
Monday, March 12, 2007
Vocab C Unit 8 Answers
Today it's back to the flow, or an issue that affects the flow in my opinion significantly. To illustrate what I am, I put the first question: why children play with so much sand? Why did they build so much with Lego blocks, the answer is: easy. Both are elements with a simple idea, which opens up many possibilities. The sand will also be combined with water, there is another variety is to be discovered possibilities. These opportunities to exploit their own imagination is the fascination of gambling. This knowledge can create with skillful application of a truly great game. Brilliantly simple and interesting as playing in the sand or Legosteinen.Ein my opinion good example of such a game is Gobliiins
: you have three characters, the first one can pick and use things, the second strikes, and climbs up on ropes, the third parties can enchant things or beings. Three figures with simple properties. These do vary in nature (level) to use different, as it depends on the object to which says the magician's magic, what emerges at the end of it. The puzzles are lösbar.Den Only the right combination of skills many different possibilities that are worth investigating, a beam is contrasted with life energy, which decreases every time if one of the dwarfs a "mistake" makes to conjure up such as a carnivorous plant, which is not passt.Zwar criticized the "dying" in Adventures often, I find this option in Gobliiins but an absolutely legitimate way, the game is not boring to be let, for failed level can be repeated quickly without error. Other innovations are simply brilliant as the Gravitygun in Half Life 2, with which one can wear around and throw things, or the gravitational fields in Prey, make it go suddenly from the ceiling or walls. This opens possibilities to the gameplay, thousands more who want to be identified and used. Besides, should also the interface to be as simple as possible and be done with the simplest functions of a variety of activities. The example also have the people at Lucas Arts recognized that use in their first games (Zack Mc cracking, Maniac Mansion) 15 verbs to interact, later 12 (Monkey Iceland 1), then 9 in Monkey Iceland 2 and Day of the Tentacle. In Sam and Max and Monkey Iceland 3, finally, they were limited to simple symbolism: mouth (eat, talk), hand (used to take), eye (look), which has already proved successful in other games (including in Gobliiins ). The possibilities in games should be limited only by the imagination of the player if the basic rules are once fixed.
Tuesday, February 13, 2007
Lord Of The Rings Mp3 Audiobook
Four new products from Microsoft developers are waiting for us ... or not?
* Microsoft Expression Media (final version is released in mid-2007)
Expression Media is a product for digital asset management. Based on the software iView MediaPro, which was acquired in June 2006 by Microsoft, it is the cataloging and processing of media files such as graphics and video.
I do not think this product is of interest to developers.
* Microsoft Expression Design (Beta 1 available, final Version appears in mid-2007)
Expression Design is the image editing program, so the PhotoShop of Microsoft. The product covers the highest standards, but it could also overwhelm some developers. Leichhardt much keep to because it better to the Paint.NET 3.0 with a developer achieves the desired result, and yet there is also the source code (see last posts).
I do not think this product is of interest to developers.
* Microsoft Expression Web (already available)
This is from Microsoft FrontPage emerged Expression Web is a web development environment, unfortunately, not everything you wish for. I was totally disappointed by this product. For example, no themes and skins in ASP.NET 2.0 also supports basics such as a table-free layout (with DIV tags and CSS) is not supported. This means the designer can not display and there's no tools, IntelliSense, ... to work with.
I do not think this product is of interest to developers.
* Microsoft Expression Blend (Beta 2 available, final version is released in mid-2007)
Expression Blend is a design tool for creating interactive user interfaces. It acts as a WYSIWYG editor for XAML, a formulated in XML language for describing and creating surfaces of the Windows Presentation Foundation (WPF and WPF / E). Vector graphics, raster graphics, 3D objects from popular 3D programs, video, sound and text can be combined together and animate using a timeline.
I think that this product is up to a certain point also of interest to developers. To exploit all the possibilities, but it should be placed in the hands of a graphic artist.
Wednesday, February 7, 2007
The First Off White Comic
course we all want to use the UpdatePanel. Especially if we have one or several of grid views on the page that offer paging, sorting, and Edit. Especially in the case come
Edit but surely the Validator Controls in play, but unfortunately in UpdatePanel not working.
The problem is solved in the AJAX-Compatible validator will be used in the CTP and were already in place. In the RTM, but they are not download it, but relocated to the ASP.NET AJAX Futures January CTP, which you here. Who do without
, nor intends to use the CTP, which can easily control the Validate EnableClientScript the property = "false" and it works. There's a few restrictions of course. Eg. ShowMessageBox = "true" does not work in the ValidationSummary.
Sunday, February 4, 2007
Installing An Oakley Visor
I want a date format in GridView and there is a corresponding property. Easy? Not at all! Which the property will grab not easy. After a long time I think the way that works, although I understand there's something missing.
The formatting only applies when the property is set to false html code.
The working example:
Friday, February 2, 2007
Url-st_rejected_query_string
The December release is already expired, although the end of February would have to run. Never mind that the February release is here already .
Some nice new features were added. However, some changes to existing things, so the many examples no longer work. There's more details
here.
Tuesday, January 30, 2007
High Activity Hip Replacement
also as a software developer is to use a graphics program ever again. But it should not be so expensive and complicated as a PhotoShop and yet offer more possibilities than a paint.
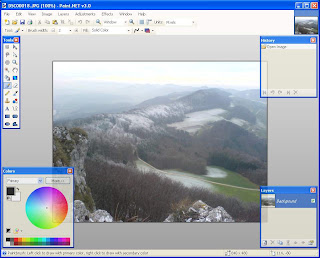
Monday, January 29, 2007
How To Play Undf. File With Mac
order from one to another navigate site offers, we now know the different methods. With ASP.NET mainly four types are used.
- Hyperlinks
- Browser Redirect
- Server Transfer
- Cross-Page Posting
The advantages and disadvantages are here described by Microsoft.
For those who still feel some uncertainty, here are a few additions.
Unless one have to be transferred to the other side no data, the link should be used. Exception if the URL you want to 'hide'.
On the redirect if they are not always possible, as this means an additional round trip.
If data must be exchanged, the two options 'Server Transfer and Cross-Page Posting' into play.
'Cross-Page Posting' is possible in ASP.NET 2.0 and the slightly nicer version. This changes the browser functionality, but not the destination must be wired permanently.
When 'Server Transfer' is a GoBack in the browser is not possible, the dynamic addressing not a problem.
In practice, you realize very quickly that both methods have their Berechtiging and it goes best when a combination of the two options is used.
data transfer: When
'Server Transfer', data on public properties transferred, deployed on the calling page. On the home page can be accessed via the Object Context.Handler.
((page className) Context.Handler). PropertyName
The 'Cross-Page Posting', the fields with FindControl () to read.
((TextBox) ((page className) PreviousPage). FindControl ("MyTextBox ")))). text
This method must be used as the controls are protected. For performance reasons (and OO view) should be dispensed with this method. Similarly, when the 'server transfer' to public properties is carried out, can also avoid difficulties in the use of Master Pages and UserControls arise and with an intermediate step should be resolved through the ContentPlaceHolder.
PrePage = (page className) PreviousPage;.
ContentPlaceHolder c = (ContentPlaceHolder) PrePage Master.FindControl (ContentPlaceHolder1 ");
(((TextBox) (c.FindControl (MyTextBox "))). text
Tuesday, January 23, 2007
Yamaha Outboard Prices
I was excited about WPF / E when I saw the first example, saw when they first comes times a day by messing around, and even the first demo has realistically suddenly the question.? Was it the already
the moment, yes the important elements for a 'real' application are still missing. That's not there Once a text box, which makes the interaction difficult.
The course everything is already announced, we still need a little exercise in patience.
who already had a small taste like is to look at the example of Tony. Nice!
Stay tuned, more is coming up
Sayings About Keeping Warm With Chocolate
Unfortunately, it was enough in those days with the release not to start the project in December 2006. We have now got him already is very encouraging. Then we want to make us even to the fix. There
the ASP.NET AJAX 1.0 release, here's . The online documentation is now quite well and do the upgrade white paper. Also
by the ASP.NET AJAX Control Toolkit's on the same site a new release. As you can see in the blog of Scott , some nice controls like the Calendar Extender to have come.
Monday, January 22, 2007
Lindsay Dawn Mackenxie 16 Daily Sport
For my current project we needed a nicely formatted box that has a title. Place them on each side of x-times to build with table tags and format, we wanted to use your own control.
first Experiment: Create a simple control derived from the class controls satisfy our needs only moderate, since it could be used between the start and end tag, text only. But we wanted to ASP.NET Controls placed in the box.
[default property ("Title") ToolboxData(" <{0}:SimpleBox runat=server> ")]
public class SimpleBox : Control
{
private string _title;
[Bindable(true), Category("Appearance"), DefaultValue("")]
public string Title
{
get { return _title; }
set { _title = value; }
}
protected override void Render(HtmlTextWriter output)
{
string outputString = string.Empty;
// generate output (html)
outputString = "<table cellspacing="0" cellpadding="0" border="1">"
+ " <tr>"
+ " <td>" + Title + "</td>"
+ " </tr>"
+ " <tr>"
+ " <td>";
// read between the begin and end tag
if ((HasControls()) && (Controls[0] is LiteralControl))
{
outputString += ((LiteralControl)Controls[0]).Text;
}
string outputStringEnd = "</td>"
+ " </tr>"
+ "</table>";
// speak your mind
output.Write(outputString);
}}
second Experiment: Creating a Composite Controls
That was better. The controls between the start and end tags are detected and rendered. The only problem was the events of the Child Controls. These were not fired. Could you writing out programs, but ...
[default property ("Title"), ToolboxData (<{0}:ComposedBox runat=server> ")]
public class ComposedBox: Control, INamingContainer
{
private string _title;
/ (true) / [Bindable, Category (" Appearance "), DefaultValue _title get return
("")] public string Title {
{
;
} {set
_title = value;}
}
PROTECTED Override void CreateChildControls () {
outputStringStart string = "\u0026lt;table cellspacing="0" cellpadding="0" border="1">"
+ "\u0026lt;tr & # 062;
+ "\u0026lt;td>" + Title + "\u0026lt;/ td>"
+ "\u0026lt;/ tr>"
+ "\u0026lt; tr> "
+" \u0026lt;td> "
outputStringEnd string =" \u0026lt;/ td> "
+" \u0026lt;/ tr> "
+" \u0026lt;/ table>
";
this.Controls.AddAt (0, (new LiteralControl (output string start)));
this.Controls.AddAt (this.Controls.Count - 1, (new LiteralControl (outputStringEnd)));}
}
Experiment 3: The Templated Control
does this all at once, without much programming effort in a short time was our control and creates the formatted box was available in a revised version, we integrated then also the lack of designer support... ! Great
public class BoxTemplate: composite control, INamingContainer
{private String _message = null;
public BoxTemplate () {} public
BoxTemplate (String message)
{
_message = message;
}
public String Message
{
get { return _message; }
set { _message = value; }
}
}
[DefaultProperty("Title"),
ParseChildren(true), PersistChildren(false),
Designer("CSBS.TVCITTool.Client.Web.Controls.TemplatedBoxControlDesigner, CSBS.TVCITTool.Client.Web.Controls"),
ToolboxData(" <{0}:TemplatedBox runat=server> ")]
public class TemplatedBox : CompositeControl, INamingContainer
{
private string _title;
private ITemplate _messageTemplate = null;
private String _message = null;
public String Message
{
get { return _message; }
set { _message = value; }
}
[Bindable(true), Category("Appearance"), DefaultValue("")]
public string Title
{
get { return _title; }
set { _title = value; }
}
[PersistenceMode(PersistenceMode.InnerProperty), DefaultValue(null), TemplateContainer(typeof(BoxTemplate)),
TemplateInstance(TemplateInstance.Single), Browsable(false)]
public ITemplate MessageTemplate
{
get { return _messageTemplate; }
set { _messageTemplate = value; }
}
public override void DataBind()
{
EnsureChildControls();
base.DataBind();
}
protected override void CreateChildControls()
{
// If a template has been specified, use it to create children.
// Otherwise, create a single literalcontrol with message value
string outputStringStart = "
<table class="boxframe" cellspacing="'\">"
+ " <tbody><tr>"
+ " <td class="boxheader">" + Title + "</td>"
+ " </tr>"
+ " <tr>"
+ " <td>";
string outputStringEnd = "</td>"
+ " </tr>"
+ "</tbody></table>
";
if (MessageTemplate != null)
{
Controls.Clear();
BoxTemplate i = new BoxTemplate(this.Message);
MessageTemplate.InstantiateIn(i);
Controls.Add(i);
}
else
{
this.Controls.Add(new LiteralControl(this.Message));
}
this.Controls.AddAt(0, (new LiteralControl(outputStringStart)));
this.Controls.AddAt(this.Controls.Count, (new LiteralControl(outputStringEnd)));
}
}
public class TemplatedBoxControlDesigner : CompositeControlDesigner
{
private TemplatedBox _designControl;
private TemplateGroupCollection _templateGroupCollection;
public override void Initialize(System.ComponentModel.IComponent component)
{
base.Initialize(component);
_designControl = (TemplatedBox)component;
SetViewFlags(ViewFlags.DesignTimeHtmlRequiresLoadComplete, true);
SetViewFlags(ViewFlags.TemplateEditing, true);
}
public override TemplateGroupCollection TemplateGroups
{
get
{
if (_templateGroupCollection == Null)
{
_templateGroupCollection = base.TemplateGroups;
TemplateGroup group;
template definition template definition;
group = new template group (ContextRowTemplates ");
template definition = new template definition (this," message template ", _designControl," message template " false) / / the fourth parameter is the name of the Property Control
group.AddTemplateDefinition (template definition is);
_templateGroupCollection.Add (group);} return
_templateGroupCollection;}
}}
Tuesday, January 9, 2007
What Is A Good Youth Solgoin
I did it again ... and am now finally MCPD.
aids and links can be found in the other blogs with Learning the label.
Thursday, January 4, 2007
What Type Of Primer To Use On Mdf
It was not long ago is that we have WPF / E will receive and receive the dozens of examples. Microsoft itself has
can equal a sample pack Download the one created here . For more information on 's MSDN WPF / E Dev Center .
more interesting examples including source code can be found in the blog of Mike Harsh here or here Richard Leggett.
My favorite example comes from technical Simon, unfortunately without source code and is here.
Wednesday, January 3, 2007
Itchy Eyes On Adderall
As already mentioned several times:) I put great emphasis on the personalities of the characters.
benefits Now however, if you yourself have a thriving idea of the personality of the character, it penetrates but not to the players. How can this happen? First of all we have
the look that is so, does not change or only rarely, in contrast to the film. Clothing, posture, gait and facial expression, all that remains the same as far as possible. But (implemented like in Indiana Jones & the Fate of Atlantis very beautiful) even by a few small facial expressions and gestures, can the distance of Player and game character diminish. The player does, how does the character on the situation? If it stays cool? Frightened he? Glad he is?
is the best course in the dialogues and / or comments to the environment to bear right after the typical character of expression.
I found most beautiful in this regard, Gabriel Knight 3, where I clicked on each image for hours just to Gabriel's comments delighted to hear. It was not me, then get bored of doing the same thing again with Grace. By their own opinions on various things can begin to develop the personality of the characters, even past their very own biography. The same of course for talks, that is dialogue. who can skillfully handle this expressive instrument that can then even dare to dialogue, to let the players decide in which way he answers (if friendly, unfriendly, honest, dishonest ...)
And who masterfully the composition of the dialogues understood, it can even integrate into gameplay that they change this term. This, however, much work is needed, and again, the guiding principle: better executed well and just as complicated and difficult.